Contoh Program Queue Dengan Linked List
Dalam contoh program berikut ini saya gunakan double linked list untuk implementasi queue. Secara umum, operasi dalam queue ada 2 yang utama yaitu enqueue dan dequeue. Enqueue berarti memasukkan item baru ke dalam antrian. Sedangkan dequeue untuk mengeluarkan item dari antrian. Queue bersifat FIFO, First In First Out. Antrian (Queue) merupakan representasi data yang hanya memperbolehkan pengaksesan data pada dua ujung. Penyisipan data dilakukan dibelakan (ekor) dan pengeluaran data dilakukan diujung (kepala). Berbeda dengan double lingked list pada praktikum 5 yang diperbolehkan mengakses data di sembarang tempat. Contoh program single linked list non circular tambah list di depan. Buatlah sebuah program yang mengimplementasikan Doubly Linked List dengan menggunakan.
I have some problems that code. I read file in code and build one stack and one queues structure. But the code wasn't run correctly.
This is Node classI used Double LinkedList
** this is stack class. **
This is Queues Class
This is Queues Class
1 Answer
Ok, so you have a couple of problems. I'm going to point out a few and let you work to fix the rest because this looks like an assignment and I don't want to do your homework for you :).
First, when you read from the file be careful not to ignore the first element:
Notice that unlike your solution I first do the push y.Push(line)
so that we don't forget to add whatever is already read into line
. Same goes for the queue file:
Just add it if it's not null
and then read the next line. You were always missing on the first element from the file.
Another problem is the Queues class (which by the way is misspelled you should replace O
with Q
). This one is not working properly because you forgot to increment and decrement the size when you insert or remove.
Notice that at the end of insert I'm increasing the size
so that the list
method doesn't throw a NullPointerException
every time we call it. Same goes for the remove
method:
Please also notice that your check before (if(head.nexttail)
) was also throwing NullPointerException
because at the beginning the head
is always null
so you cannot access the next
member. Finally I've made a small improvement to the list
method as well so that we return earlier:
Notice the return
if the Queue is empty, otherwise we will attempt to do tail.getNext()
which will always throw a NullPointerException
.
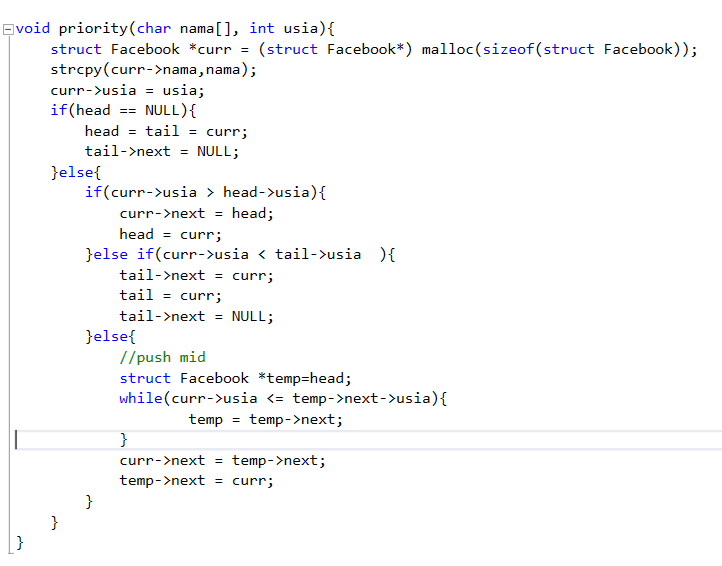
Some important thoughts about the code in generalPlease avoid weird naming. Why Queues? There is just one so it should be Queue.Please avoid weird variable names. Your code is not just for you, chances are someone else might need to read and it gets hard to know who it is s, y, k, fwy and fwk
. Why not naming them like this:
And the same goes for methods . Why Push
, Pop
and Top
are the only methods that start with upper-case letter ? If you don't agree with the standard Java naming convention that is fine but at least be consistent :).
Tema logo club bola. May 20, 2016 - Football logo club Keyboard can be downloaded and installed on android devices supporting 9 api and above. Please note that we provide original and pure apk file and provide faster download speed than Football logo club Keyboard apk mirrors.. You could also download apk of. Oct 10, 2015 - Tema keyboard bola ini akan menampilkan nama club bola dunia yang. Lagi logo bola dunia yang biasa anda tampilkan di keyboard anda. Find your perfect design for the Futsal Logo Tournament at Vecteezy! Over 1000 free futsal clipart & icons to perfect your contest submission. Are you looking for Bola Logo vectors or photos? We have 2497 free resources for you. Download on Freepik your photos, PSD, icons or vectors of Bola Logo. This Football Team Jersey World Theme is specially designed free for CM Launcher which has beautiful world football team jersey wallpapers, green football.
Try the suggested improvements and see how your program it's working. I'm almost sure there are more problems with it. If you can't figure them out yourself leave a comment and I will help you. Good luck!
Not the answer you're looking for? Browse other questions tagged javadata-structures or ask your own question.
/*Queue - Linked List implementation*/ |
#include<stdio.h> |
#include<stdlib.h> |
struct Node { |
int data; |
struct Node* next; |
}; |
// Two glboal variables to store address of front and rear nodes. |
struct Node* front = NULL; |
struct Node* rear = NULL; |
// To Enqueue an integer |
voidEnqueue(int x) { |
struct Node* temp = |
(struct Node*)malloc(sizeof(struct Node)); |
temp->data =x; |
temp->next = NULL; |
if(front NULL && rear NULL){ |
front = rear = temp; |
return; |
} |
rear->next = temp; |
rear = temp; |
} |
// To Dequeue an integer. |
voidDequeue() { |
struct Node* temp = front; |
if(front NULL) { |
printf('Queue is Emptyn'); |
return; |
} |
if(front rear) { |
front = rear = NULL; |
} |
else { |
front = front->next; |
} |
free(temp); |
} |
intFront() { |
if(front NULL) { |
printf('Queue is emptyn'); |
return; |
} |
return front->data; |
} |
voidPrint() { |
struct Node* temp = front; |
while(temp != NULL) { |
printf('%d',temp->data); |
temp = temp->next; |
} |
printf('n'); |
} |
intmain(){ |
/* Drive code to test the implementation. */ |
// Printing elements in Queue after each Enqueue or Dequeue |
Enqueue(2); Print(); |
Enqueue(4); Print(); |
Enqueue(6); Print(); |
Dequeue(); Print(); |
Enqueue(8); Print(); |
} |
commented May 25, 2015
Thnx |
commented Oct 30, 2015
There is a mistake in dequeue method .you need to do temp = temp->next; |
commented Apr 4, 2016
Shouldn't you return something with the Dequeue function? |
commented Jul 23, 2016
Why have you used the Front function? It's never really called. Please explain the purpose of this function definition. |
commented Jul 23, 2016
@MRSharff - The purpose of dequeue function is to delete a node from the linked list. It has nothing to do with returning something. |
commented Nov 6, 2016
Thanks a lot for this sample code. |
commented Nov 15, 2016 • edited
edited
Hey, There is a typo in Front function, when frontNULL - > return -1 or something instead of nothing. |
commented Mar 14, 2017
Your code is very helpful here is another example hope it adds to your concept. |
commented Apr 28, 2017
There is a simpler one here |
commented Nov 27, 2017 • edited
edited
commented Dec 20, 2017
what we must do if the Data in our struct was int and string (number and name of student) |
commented Dec 25, 2017
can anyone tell me what is the use of the function int Front in this code?I am confused. |
commented Jan 29, 2018
@labeelola This is when you want to view, the latest value in front of the queue. This is not called, but that's not a problem, you can call it anywhere. It is just to view. |
commented Mar 21, 2018
Hey guys, Please help me to complete my Assignment, I dont know much about C Programming
Remark: ADT Queue is 'First in First Out'. enqueue - always add a new node at the back of the queue. dequeue - delete the head of the queue. |
commented Aug 10, 2018
Thank you so much |
commented Aug 26, 2018
sir make videos on other topics also,i observed that u r not uploading videos from past 2-3 years ,why sir? |
commented Oct 13, 2018
THANKS ..IT'S SIMPLE AND COOL .. |
commented Jan 3, 2019
Its to get the first element of the Queue(He didn't call it though) |
commented Feb 11, 2019
If anyone tried with local front and rear variables in main method ! |
commented Feb 11, 2019
in the Dequeue function when the second if(frontrear) runs we have to free the space of node pointed by front previously i.e first node is the one when front and rear are equal if we modify the pointer front and rear to NULL we are wasting the memory of first node that was created |